Initiate a payment
You can build the Pay by Bank payment experience into your existing checkout flow. For a complete payment experience, the user flow runs in this order:
- Your customer selects Pay by Bank as a payment option.
- You initiate the payment using
POST /payments
. - The
POST
request returns an ID and a link which you display to your customer. This link can also be used to automatically open the Pay by Bank screens. - Your customer gives their permission through the website flow. There are no additional API calls to make during this process, the UX guides the customer through the payment process.
- Once payment is complete, your customer is able to return to your checkout screens.
- Using the ID returned in the original request, you send a request to
POST payments/{id}/acknowledge
- Display the payment acknowledgement details to your customer.
- Retrieve payments with
GET /payments/{id}
Initiate a payment
To initiate a payment, you need to send a POST /payments
request.
In this request, you must include:
debtor
information - the name of the party making the payment.market
information - the ISO code for the market where the payment is being made.creditor
information - the party receiving the payment. Including name, account details and type.reference
- your unique ID for the payment.paymentAmmount
- the amount being paid and the currency.paymentType
- the type of payment being made. This can beRTP
,LOW_VALUE_RETAIL
orHIGH_VALUE_BUSINESS
.
curl --request POST \
--url https://api-mock.payments.jpmorgan.com/tsapi/pay-by-bank/v2/payments \
--header 'Accept: application/json' \
--header 'Content-Type: application/json' \
--header 'Idempotency-Key: ' \
--data '{
"debtor": {
"name": "Bala Thandapani"
},
"market": "GB",
"creditor": {
"name": "Newgen Media LLC",
"account": {
"accountId": "06090887654321",
"accountIdType": "SORT_CODE"
}
},
"reference": "subscription - #78004300",
"paymentType": "RTP",
"redirectURL": "https://api.newgenmediacorp.co.uk/pay-by-bank/callback",
"paymentAmount": {
"value": "100.00",
"currency": "GBP"
}
}'
{
"id": "1b036f9c-8c84-4ce6-b1dd-5979472945a1",
"debtor": {
"name": "Bala Thandapani"
},
"market": "GB",
"status": "NONE",
"creditor": {
"name": "Newgen Media LLC",
"account": {
"accountId": "11223387654321",
"accountIdType": "SORT_CODE"
}
},
"createdAt": "2023-08-20T13:31:25Z",
"reference": "subscription - #78004300",
"paymentType": "RTP",
"redirectURL": "https://api.newgenmediacorp.co.uk/pay-by-bank/callback",
"paymentAmount": {
"value": "100.00",
"currency": "GBP"
},
"paymentQRCode": "https://images.newgenmediacorp.co.uk/image/1b036f9c-8c84-4ce6-b1dd-5979472945a1",
"paymentLinkURL": "https://payments-link.jpmorgan.com/1.0/pay/direct?client_id=9cd2e1c9527e4e18ad4756393e09cb2c&redirect_uri=https://api.newgenmediacorp.co.uk/pay-by-bank/callback&market=DE&payment_request_id=1b036f9c-8c84-4ce6-b1dd-5979472945a1",
"statusUpdatedAt": "2023-08-20T13:31:25Z"
}
Display the payment flow
In the POST /payments
200 response, you can see the values for the QR code and payment link that should be made available in the payment experience:
paymentQRCode
paymentLinkURL
Use the link in paymentLinkURL
to open a browser frame to the payment session. This can be customer activated, or simply opened automatically when you get the successful response to your POST
request.
If the payment is being made on a desktop or anything other than a mobile device, you can choose to display the QR code using the link provided in paymentQRCode
.
Keep the window open while your customer is guided through the process.
Acknowledge payment
Once the payment flow has been completed, your browser frame should close, and your customer returned to your payment experience.You can now confirm that the payment has been made using POST /payments/{id}/acknowledge
.
curl --request POST \
--url https://api-mock.payments.jpmorgan.com/tsapi/pay-by-bank/v2/payments/id/acknowledge \
--header 'Accept: application/json' \
--header 'Content-Type: application/json'
{
"id": "1b036f9c-8c84-4ce6-b1dd-5979472945a1",
"debtor": {
"name": "Bala Thandapani",
"account": {
"accountId": "06080912311871",
"accountIdType": "SORT_CODE"
}
},
"market": "GB",
"status": "SENT",
"creditor": {
"name": "Newgen Media LLC",
"account": {
"accountId": "11223387654321",
"accountIdType": "SORT_CODE"
}
},
"createdAt": "2023-08-20T13:31:25Z",
"reference": "subscription - #78004300",
"paymentType": "RTP",
"redirectURL": "https://api.newgenmediacorp.co.uk/pay-by-bank/callback",
"acknowledged": "ACKNOWLEDGED",
"paymentAmount": {
"value": "100.00",
"currency": "GBP"
},
"paymentQRCode": "https://images.newgenmediacorp.co.uk/image/1b036f9c-8c84-4ce6-b1dd-5979472945a1",
"paymentLinkURL": "https://payments-link.jpmorgan.com/1.0/pay/direct?client_id=9cd2e1c9527e4e18ad4756393e09cb2c&redirect_uri=https://api.newgenmediacorp.co.uk/pay-by-bank/callback&market=DE&payment_request_id=1b036f9c-8c84-4ce6-b1dd-5979472945a1",
"statusUpdatedAt": "2023-08-20T13:31:25Z"
}
You can see in this response that the payment request has a status
of SENT
and and acknowledged
value of ACKNOWLEDGED
.
From your customer's perspective, this means the payment has been accepted and they can move to the next phase of your checkout process.
Get details of a payment
You can request details of any payment using the paymentId
in a request to GET /payments/{id}
.
curl --request GET \
--url https://api-mock.payments.jpmorgan.com/tsapi/pay-by-bank/v2/payments/id \
--header 'Accept: application/json'
{
"id": "1b036f9c-8c84-4ce6-b1dd-5979472945a1",
"debtor": {
"name": "Bala Thandapani",
"account": {
"accountId": "06080912311871",
"accountIdType": "SORT_CODE"
}
},
"market": "GB",
"status": "SENT",
"creditor": {
"name": "Newgen Media LLC",
"account": {
"accountId": "11223387654321",
"accountIdType": "SORT_CODE"
}
},
"createdAt": "2023-08-20T13:31:25Z",
"reference": "subscription - #78004300",
"paymentType": "RTP",
"redirectURL": "https://api.newgenmediacorp.co.uk/pay-by-bank/callback",
"acknowledged": "ACKNOWLEDGED",
"paymentAmount": {
"value": "100.00",
"currency": "GBP"
},
"paymentQRCode": "https://images.newgenmediacorp.co.uk/image/1b036f9c-8c84-4ce6-b1dd-5979472945a1",
"paymentLinkURL": "https://payments-link.jpmorgan.com/1.0/pay/direct?client_id=9cd2e1c9527e4e18ad4756393e09cb2c&redirect_uri=https://api.newgenmediacorp.co.uk/pay-by-bank/callback&market=DE&payment_request_id=1b036f9c-8c84-4ce6-b1dd-5979472945a1",
"statusUpdatedAt": "2023-08-20T13:31:25Z"
}
Reference
These are the data fields returned in the response to successful POST /payments
and GET /payments/{id}
requests:
Field Name | Description |
---|---|
value | The value representation of a monetary amount. The value should be more than 0.01 with maximum of two decimal places allowed. |
currency | ISO 4217 Alpha-3 Currency Code |
paymentType | The type of payment |
market | ISO 3166-1 alpha-2 country code for the market where the payment is initiated |
redirectURL | An optional re-direct URL of the merchant where the end-user will be redirected to once they have successfully authorized the payment with their bank or financial institution. |
name | Name of the debtor |
accountId | Debtor account number as identified by the bank or financial institution |
accountIdType | The account identifier type |
name | Name of the creditor |
accountId | Creditor account number as identified by the bank or financial institution |
accountIdType | The account identifier type |
reference | A free text field that can be used to provide a meaningful reference for a payment transaction |
id | A unique identifier of the payment |
status | The status of the submitted payment request |
statusUpdatedAt | The last updated date and time of the payment status in ISO 8601 format |
statusMessage | A textual description of the status |
createdAt | Indicates when the Payment request was created in the system. Follows ISO date time format |
paymentLinkURL | An HTTPS URL to make the payment |
paymentQRCode | An HTTPS URL to download the QR code image to make the payment |
acknowledged | Indicates whether the payment has been acknowledged or not by the API caller. |
providerName | Pre-filled provider (FInancial Institution) name which triggers the skipping of the Bank selection experience |
Sequence diagram of API requests for payment initiation
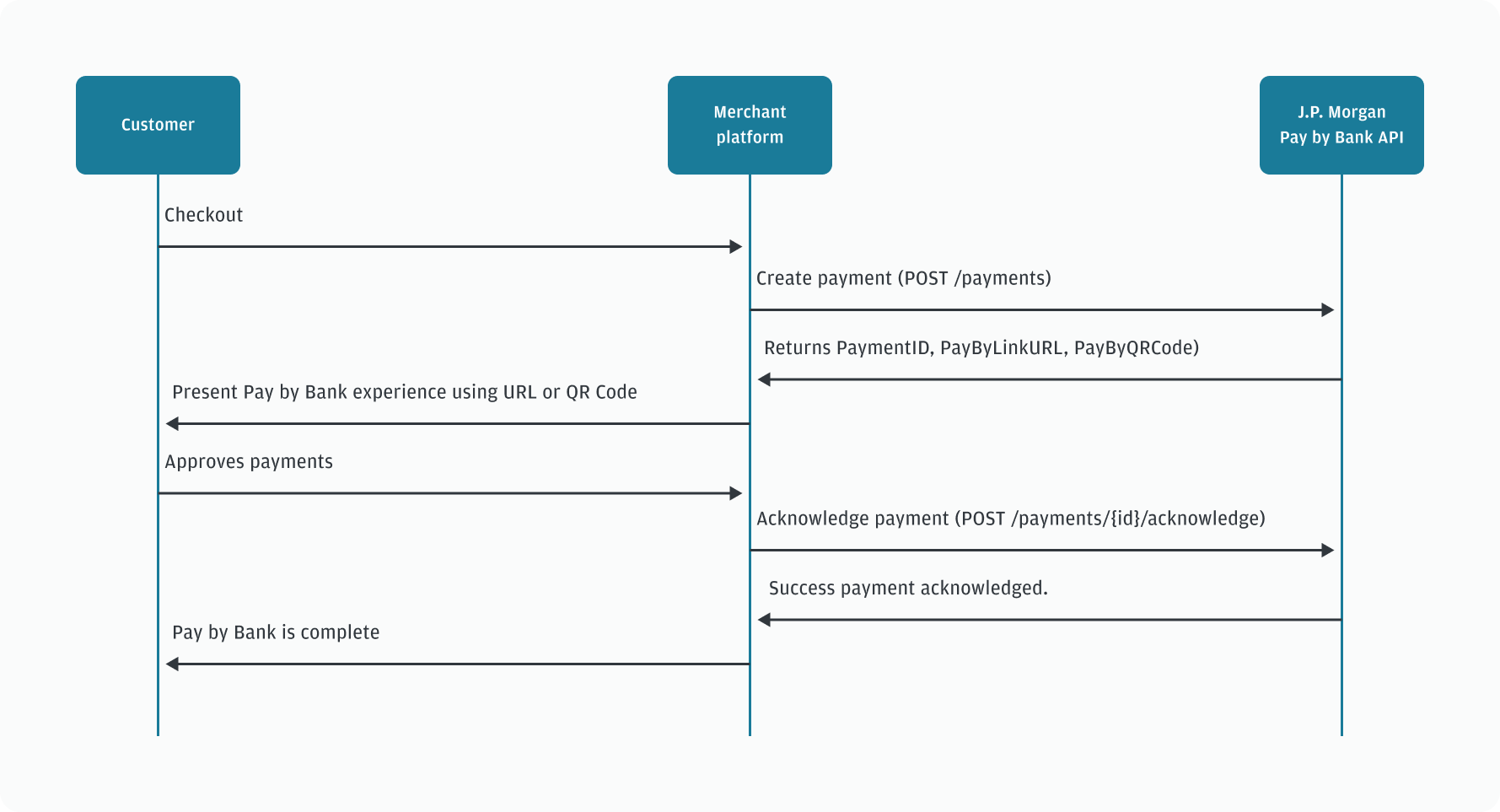