Drop-in UI
Drop-in UI is a complete, ready-made payment UI that offers a quick and easy way to securely accept payments. You can customize your Checkout form to fit stylistically into your current checkout experience by using the Checkout Settings page in the Commerce Center portal, or by using JavaScript to modify basic styles or the full theme object.
Before you begin
To use drop-in UI, you must first Set up a drop-in UI.
How it works
Here's a diagram that illustrates the Drop-In user interface (UI) process flow to help you better understand the flow of information between the components.
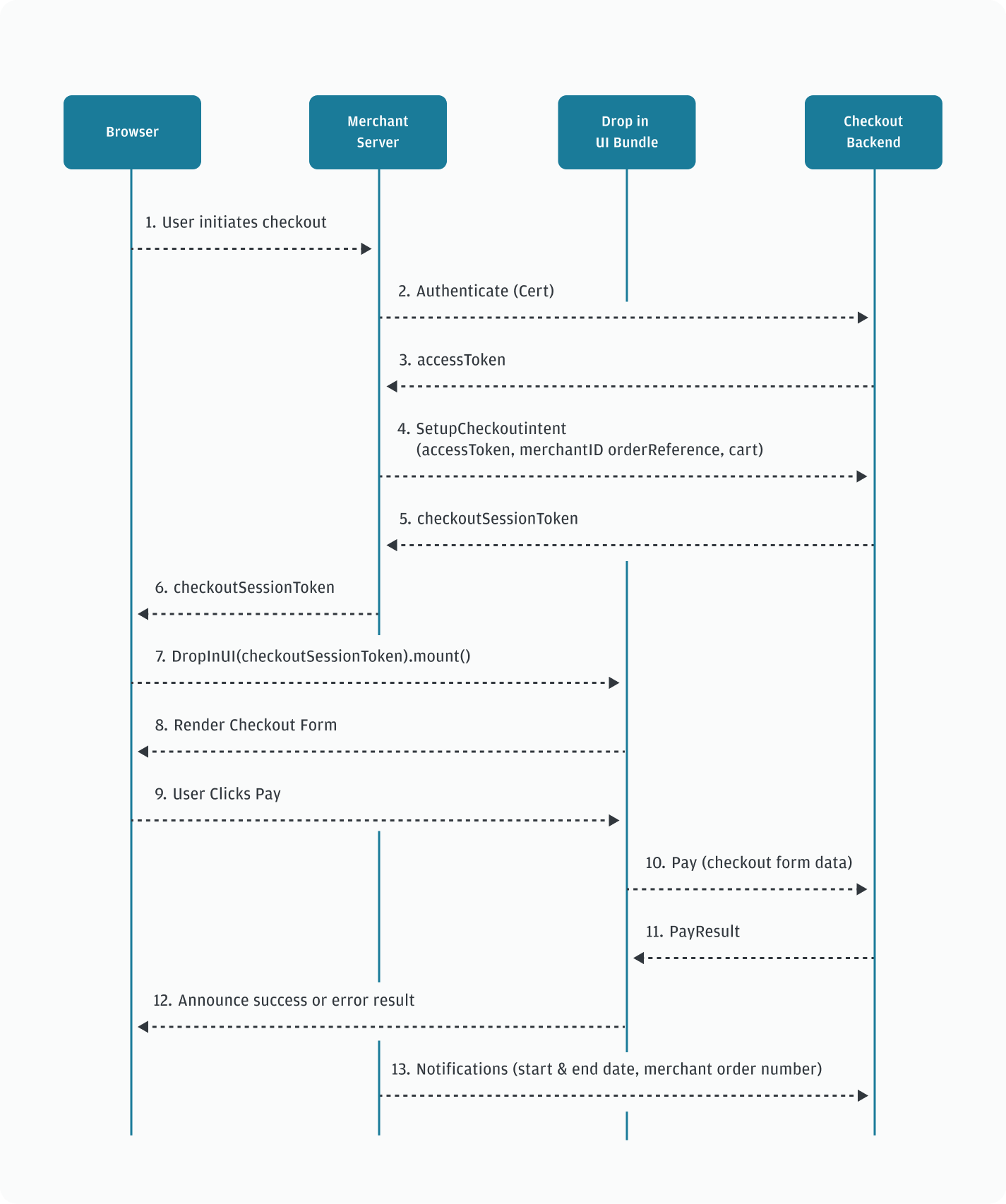
- The browser initiates a checkout with the merchant server.
- The merchant server sends an authentication request via the Checkout backend.
- If authentication is successful, an
accessToken
is sent in response to the merchant server. - The merchant server creates a checkout session by sending a
POST
request to the/checkout/intent
endpoint, using the accessToken (bearer token), the merchant ID, an order reference, and a cart object. - Checkout backend sends the
checkoutSessionToken
as a response to the merchant server. - The merchant server sends checkoutSessionToken to the browser.
- The merchant webpage loads the Drop-In UI bundle and initializes it with the checkoutSessionToken.
- Drop-In UI renders the Checkout form on the browser.
- The consumer clicks Place Order / Pay (configurable) on the pay form.
- Drop-In UI initiates Pay (checkout form data) with the Checkout backend.
- Checkout backend sends the payResult to the Drop-In UI bundle.
- Drop-In UI bundle sends a success or failure response to the browser to relay a status to the consumer.
- Request transaction details from Checkout notifications (start & end date, merchant order number).
Customization
Configure and customize your Checkout page using one of the following methods:
- Checkout Settings page in the Commerce Center
- JavaScript theme objects
Configure using Commerce Center
You can use the Checkout Settings page in the Commerce Center portal to configure the Checkout experience.
Perform the following steps to configure Checkout settings:
- Log in to the Commerce Center.
- Go to Settings.
- Click on Checkout from the drop-down list.
- Select the configuration settings as per your business needs.
- Once you are done, click Publish.
Configure using JavaScript
User the following JavaScript functions within the HTML script tag to customize your Checkout page.
Basic styles
The DropInUI function accepts a theme override object that can be used to customize the colors, borders, fonts, and density within your Checkout form as shown:
hf = new DropInUI({
checkoutSessionToken: getCheckoutToken(),
themeValueOverrides: {
color: {
brand: "#CC0000",
border: "#CC0000",
interactive: "#AA0000"
},
borderRadius: {
"form": "0",
"button": "25px"
},
fontFamilies: {
"h1": "Open Sans",
"h2": "Open Sans"
},
},
});
hf.mount("root");
Colors
Customize the colors that are used to create the application’s color palette. These can be in Hue-Saturation-Light-Alpha (HSLA) or Hexadecimal (HEX) format. The colors in the following example calculate additional colors that are used in the application.
const theme = {
"color": {
"brand": "hsla(232, 60%, 47%, 1)",
"interactive": "hsla(206, 91%, 40%, 1)",
"pageBackground": "hsla(220, 60%, 99%, 1)",
"containerBackground": "hsla(0, 0%, 100%, 1)",
"formBackground": "hsla(0, 0%, 100%, 1)",
"primaryText": "hsla(231, 8%, 17%, 1)",
"secondaryText": "hsla(215, 13%, 56%, 1)",
"tertiaryText": "hsla(214, 20%, 73%, 1)",
"border": "hsla(240, 7%, 42%, 1)",
"divider": "hsla(240, 7%, 42%, 1)",
"critical": "hsla(4, 79%, 48%, 1)",
"positive": "hsla(124, 100%, 27%, 1)"
}
};
Borders
The border radius controls the radius for form fields and buttons. The form field radius also applies to the boxes that outline sections of the application.
const theme = {
"borderRadius": {
"form": "8px",
"button": "0px"
}
};
Fonts
Font families can be used from the given list of fonts.
- The fontSizes, lineHeight, and letterSpacings attributes are defined by the tag that will use the property on the page. These can be defined in pixel, em, or rem.
- The fontWeights attribute is also defined by the element that uses them. The fontWeight attribute can accept string values of whole hundred numbers from 100 to 900.
- The fontFamily attribute allows different font families to be applied to the given element types.
const theme = {
"typography": {
"fontFamily": "Inter"
},
"fontSizes": {
"h1": "2rem",
"h2": "1.5rem",
"h3": "1.25rem",
"h4": "1rem",
"body": "1rem",
"caption": "0.875rem"
},
"lineHeights": {
"h1": "2.5rem",
"h2": "1.995rem",
"h3": "1.75rem",
"h4": "1.5rem",
"body": "1.5rem",
"caption": "1.37375"
},
"letterSpacings": {
"h1": "-0.03rem",
"h2": "-0.015rem",
"h3": "0.00625rem",
"h4": "0rem",
"body": "0rem",
"caption": "0.01225rem"
},
"fontWeights": {
"h1": "600",
"h2": "500",
"h3": "500",
"h4": "500",
"body": "400",
"caption": "400"
},
"fontFamilies": {
"h1": "Inter",
"h2": "Inter",
"h3": "Inter",
"h4": "Inter",
"body": "Inter",
"caption": "Inter"
}
};
Density
Density controls the spacing around elements. densityType can be compact, default, or spacious.
const theme = {
"densityType": "compact" | "default" | "spacious"
};
Full theme object
A full theme object is available to modify and pass in the themeValueOverrides property when creating a new DropInUI instance.
const theme = {
"color": {
"brand": "hsla(221, 83%, 53%, 1)",
"interactive": "hsla(221, 83%, 53%, 1)",
"pageBackground": "hsla(210, 40%, 98%, 1)",
"containerBackground": "hsla(0, 0%, 100%, 1)",
"formBackground": "hsla(0, 0%, 100%, 1)",
"primaryText": "hsla(217, 33%, 17%, 1)",
"secondaryText": "hsla(215, 16%, 48%, 1)",
"tertiaryText": "hsla(214, 20%, 73%, 1)",
"border": "hsla(214, 21%, 60%, 1)",
"divider": "hsla(214, 32%, 91%, 1)",
"critical": "hsla(345, 83%, 41%, 1)",
"positive": "hsla(163, 94%, 24%, 1)"
},
"borderRadius": {
"form": "4px",
"button": "4px"
},
"densityType": "default"
"typography": {
"fontFamily": "Inter"
},
"fontSizes": {
"h1": "2rem",
"h2": "1.5rem",
"h3": "1.25rem",
"h4": "1rem",
"body": "1rem",
"caption": "0.875rem"
},
"lineHeights": {
"h1": "2.5rem",
"h2": "1.995rem",
"h3": "1.75rem",
"h4": "1.5rem",
"body": "1.5rem",
"caption": "1.37375"
},
"letterSpacings": {
"h1": "-0.03rem",
"h2": "-0.015rem",
"h3": "0.00625rem",
"h4": "0rem",
"body": "0rem",
"caption": "0.01225rem"
},
"fontWeights": {
"h1": "600",
"h2": "500",
"h3": "500",
"h4": "500",
"body": "400",
"caption": "400"
},
"fontFamilies": {
"h1": "Inter",
"h2": "Inter",
"h3": "Inter",
"h4": "Inter",
"body": "Inter",
"caption": "Inter"
},
}
Mount and unmount
After the drop-in UI instance is created, you can mount and unmount the drop-in UI to your webpage by using the following commands.
Command | Description |
---|---|
myDropIn.mount("target-element-id") |
Use the mount command and provide the ID of a HTML element that drop-in UI will use to render the contents. |
myDropIn.unmount() |
Use the unmount command to remove the mounted drop-in UI which will empty the assigned HTML element and remove any subscribers. |
var myDropIn = myDropInUI({
checkoutSessionToken: "getCheckoutToken()",
});
myDropIn.mount("target-element-id");
// ready to remove myDropInUI
myDropIn.unmount()
Drop-in UI event bus
The goal of the event bus is to provide you access to information about what is occurring inside the Drop-in UI. These can be lifecycle events, errors, or other events.
Subscribe to these lifecycle event announcements using the subscribe() built-in function in the Drop-in UI mount. To unsubscribe, use the returned reference from subscribe(), which in the following example is unsubscribeRef().
var myDropIn = myDropInUI({
checkoutSessionToken: "getCheckoutToken()"checkout-sessiontoken",
});
myDropIn.mount("target-element-id");
const unsubcribeRef = myDropIn.subscribe((announcement) => {
if (announcement.namespace === "payment" && announcement.level === "info") {
if (announcement.message === "PaymentSuccess") {
handlePaymentSuccess(announcement);
}
if (announcement.message === "PaymentUnsuccessful") {
handlePaymentFailure(announcement);
}
}
if (announcement.namespace === "payment" && announcement.level === "error") {
handlePaymentFailure(announcement);
}
}),
//Ready to unsubscribe
unsubscribeRef()
Using the announcement, you can choose to display a certain message, redirect consumers, and perform many other actions based on your requirements.
Announcement details
Announcements are sent to all subscribers and have four properties: level, namespace, message, and payload.
level: "error" | "warn" | "info";
namespace: string;
Message: PaymentSuccess;
Payload:
payment_response
{
"status": "STATUS_SUCCESS",
"maskedPan": "************4242",
"cardExpirationMonth": 12,
"cardExpirationYear": 2026,
"approvalCode": "tst794",
"paymentGatewayTransactionId": "66D8977818CC7D2000000C150000DE3F415653F6"
};
Level
Level is provided to indicate the type of announcement.
Level Type | Description |
---|---|
error | Used for sending errors that will break or prevent the payment process. They may not be recoverable and will need to be handled. |
info | Used for sending informational events. These should have no impact on the user experience. The main goal is to allow the merchant website to know more about when the application is ready and when the payment is complete. |
warn | Used for sending warnings that may impact the user experience but should not prevent the payment process from working. |
command | Used for handling announcements generated by the client's actions requesting for something to be processed. |
Namespace
Namespace is provided to indicate where the event originated.
Message
Message is a string description of the event. These are fixed per event and can be directly matched against.
Level | Namespace | Message | Description |
---|---|---|---|
error | unknown | Unknown | An unclassified error occurred and propagated up to the root of the drop-in UI. This should not happen, but it may indicate a larger problem with the web page. |
error | unknown | UnknownError | An uncaught error occurred and propogated up to the root. The UI has stopped rendering the form and is showing an error message. |
error | payment | PaymentError | The payment request has failed. It may be recoverable in some scenarios. There should be more information in the payload under the error key. |
error | payment | CreditCardEncryptionError | The credit card encryption process was not successful. The required information to encrypt data is invalid, or the browser doesn't support encryption. |
error | payment | SepaEncryptionError | The SEPA encryption process was not successful. The required information to encrypt data is invalid, or the browser doesn't support encryption. |
error | payment | EcpAccountNumberEncryptionError | The ECP account number encryption process was not successful. The required information to encrypt data is invalid, or the browser doesn't support encryption. |
error | payment | EcpRoutingNumberEncryptionError | The ECP routing number encryption process was not successful. The required information to encrypt data is invalid, or the browser doesn't support encryption. |
info | payment | PaymentSuccess | The payment is successful. The merchant can choose what to do next. |
info | payment | PaymentUnsuccessful | The payment has failed. You can choose what should happen next. This may be from a decline, or another reason. The consumer can attempt a resolution. |
info | payment | PaymentValidationInProgress | The payment is in progress. An additional step to validate the payment has occurred. Example: 3DS validation. |
info | payment | PaymentValidationFailed | The validation failed when submitting the payment form. The announcement does not include what failed. |
info | render | MountSuccess | The form has been successfully displayed to the consumer. |
info | render | InitialLoadComplete | The form has received the information it need to render and should be visible to the user. |
Payload
Payload is an object of key and value pairs. This can vary based on message value.