WebSocket basics
5 December 2024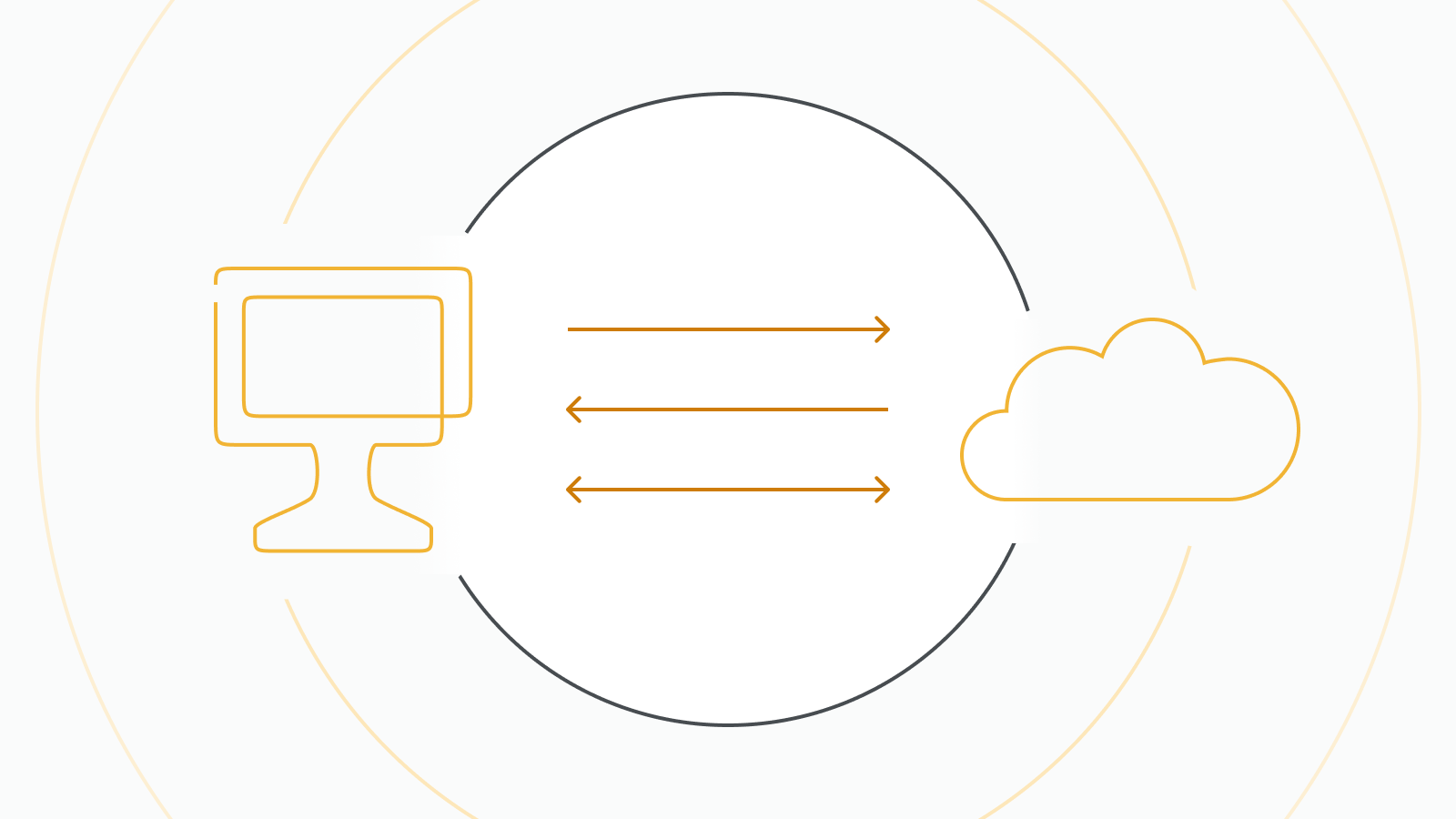
A case for WebSocket
When we talk about APIs today, most people think of REST. There are other protocols to fit certain requirements, but REST APIs are popular in web-based applications. Even at J.P. Morgan Payments, we decided to primarily support REST APIs. This is because the REST framework is simple and follows HTTP protocols, meaning REST will integrate with programs already using the internet.
That said, there are still strong arguments and use cases for other kinds of APIs.
REST APIs shine for stateless, synchronous data flows. In other words, a client makes a self-contained request and waits for a response before moving on, and that transaction is not concerned with state management.
But what about when stateful, asynchronous data flows are required? Technically, REST can be used asynchronously, but REST is inherently stateless. Protocols that support asynchronous stateful flows include HTTP2/3 and WebSocket. There are pros and cons for each of these options, but we’ll be looking at WebSocket in this post, as that’s what our In-Store Payment Terminal Application solution leverages. Other scenarios where WebSocket should be considered include the following:
- When you would rely on polling with REST
- If the order of events is important to you
- When you want to reduce latency (either eliminating latency caused by high traffic, or when near-zero latency is required for real-time scenarios)
The WebSocket protocol is an events-based messaging system over a full-duplex (data transmission goes both ways between client and server) TCP/IP connection. Just like the Fetch API in JS is a specification by which clients can leverage HTTP, the WebSocket specification provides access to the WebSocket protocol.
Client-server connection
Whether communicating via REST or WebSocket requests, APIs connect a client (e.g., a web browser) to a server to access and/or modify data on that server.
To better understand this, let’s use our In-Store solution as an example. The Payment Terminal Application expects a client-server relationship with the point of sale (POS), wherein the Payment Terminal Application is the server and the POS is the client. A POS must follow the API Messaging Protocol to communicate with the Payment Terminal Application.
The POS chooses the appropriate length of time to keep the WebSocket connection open, and opens and closes the connection as needed. When the POS maintains a connection over multiple transactions, the connection should be refreshed periodically (e.g., once every hour). The interval depends on business logic needs, and longer intervals are acceptable. Note that no connection will stay alive for longer than 24 hours. This is due to the PCI DSS requirement for Payment terminals to reboot every 24 hours (at a minimum).
To begin a payment transaction flow, the POS client makes a request to the Payment Terminal Application. The Payment Terminal Application manages the logic of gathering card data and other payment details and then passes these details to our payment host for approval.
How to manage a broken WebSocket connection
Messages can only be exchanged while a connection is open. The client can decide to purposefully close a connection, but sometimes a connection will unexpectedly break. The most common causes for broken connections are network connectivity timeouts and endpoint reboots (planned or unplanned).
To handle a broken connection, logic is necessary on both the client and server side.
In the case of our In-Store Payment Terminal Application solution, the POS client manages the WebSocket connection as the client connection. If a WebSocket connection is closed, then the POS client must reconnect to the Payment Terminal Application. If the connection drops during a payment transaction, perform the following steps to determine the status of the previous transaction:
- POS client reconnects to Payment Terminal Application
- To obtain the approval status of the previous transaction, perform the Get Last Transaction command
- Confirm WebSocket connection is active
Note that Payment Terminal Application will attempt to automatically reverse a transaction if the connection is broken during the connection to the host.
To maintain awareness of the health of your persistent WebSocket connection, perform a health check. Some APIs provide pings and pongs for this purpose. You can also implement your own health check, also known as a heartbeat, at a set interval (between one and five minutes) or prior to the start of any sequence of messages.
In the case of our Payment Terminal Application API, you should use the Get Information command (a lightweight command that returns useful data to the POS) to implement your own health check.
WebSocket resources
WebSockets are one of the most useful protocols for receiving and sending real-time data. Now that you are primed up on the protocol, here are some further resources:
- The WebSocket API (MDN doc on the JavaScript WebSocket API)
- Python WebSocket (Python package to create and manage WebSocket connections)
Some of the J.P. Morgan Payment APIs use WebSockets to transmit data involved in a payment. Explore the following docs to learn more: